This example shows how to use the core of YUI.
for
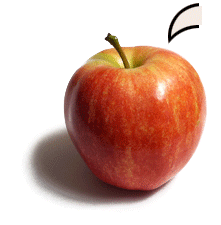
for
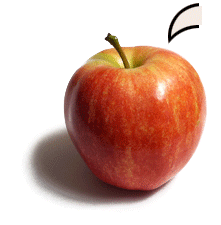
Setting up the YUI Instance
Here we will create our YUI
instance, loading node
so we can work with DOM elements in the example.
YUI().use('node', ...
Using the callback
The use
method will dynamically fetch anything required for Node
if it isn't already on the page.
If dynamic loading is required, the last parameter supplied to use
should be a function to execute when
the load is complete. This function will be executed whether or not dynamic loading is required, so it
is the preferred pattern for using YUI
.
YUI().use('node', function(Y) ...
The function is supplied a reference to the YUI
instance, so we can wrap all of our implementation code inside of
the use
statement without saving an external reference to the instance somewhere else.
Now that we know all of the modules are loaded, we can use Node
to update DOM nodes in many ways.
Here's a simplified version of the fruit example above.
<span> Box 1 </span> <span class="bob"> Box 2 </span> <script> YUI().use('node', function(Y){ // change the style of just the DOM node with the class "bob" Y.one('.bob').setStyle('backgroundColor', '#FFD7AA'); }); </script>
Full Source
Here's the full source of the fruit example.
<style> .example { position: relative; font-family: georgia; height: 260px; } .example p { margin: 0; } .example .count { font-size: 250%; display: inline-block; line-height: 0.8em; zoom: 1; *display: inline; /* IE < 8: fake inline-block */ width: 0.6em; vertical-align: baseline; font-family: verdana; } .example em { font-size: 170%; font-weight: bold; text-shadow: 0 0 4px rgba(110, 132, 57, 0.75); display: block; font-style: normal; } .example .fruit { position: absolute; top: 21px; left: 200px; border: solid 3px #000; background-color: #F4EBE2; -moz-border-radius: 20px; -webkit-border-radius: 20px; border-radius: 20px; padding: 0.6em 0.6em 0.9em; text-align: center; box-shadow: 3px 4px 10px rgba(0, 0, 0, 0.3); font-size: 125%; } .example .changer { left: 464px; } .example .fruit img { position: absolute; top: 35px; left: -202px; } </style> <div class='fruit'> <div class="speech">We're twins<br> for <div class="count">5</div> sec. </div> <img src="../assets/yui/images/apple.png" width="221" height="225"/> </div> <div class='fruit changer'> <div class="speech">We're twins<br> for <div class="count">5</div> sec. </div> <img src="../assets/yui/images/apple.png" width="221" height="225"/> </div> <script> YUI().use('node', function(Y) { var count = 6, // seconds for timer count down changer = Y.one('.changer'), // just the node to change counters = Y.all('.fruit .count'); // counter numbers on both fruits var doChange = function(){ count -= 1; // decrement the seconds count counters.setHTML(count); // update the number of seconds by setting the contents of the node // when it gets to 0 seconds, make changes to just the node with class "changer" if(count < 1){ // set the "src" attribute of one image to an orange Y.one('.changer img').setAttribute('src', '../assets/yui/images/orange.png'); // change the contents of one speech bubble Y.one('.changer .speech').setHTML("No, I'm from <em>Florida!</em>"); // change several styles together changer.setStyles({ fontSize: '150%', color: '#EA6500', backgroundColor: '#FFD7AA' }); return; // bypass the setTimeout and don't call the function again } setTimeout(doChange, 1000); } doChange(); }); </script>