Getting Started
To include the source files for Console and its dependencies, first load the YUI seed file if you haven't already loaded it.
<script src="http://yui.yahooapis.com/3.8.0/build/yui/yui-min.js"></script>
Next, create a new YUI instance for your application and populate it with the
modules you need by specifying them as arguments to the YUI().use()
method.
YUI will automatically load any dependencies required by the modules you
specify.
<script> // Create a new YUI instance and populate it with the required modules. YUI().use('console', function (Y) { // Console is available and ready for use. Add implementation // code here. }); </script>
For more information on creating YUI instances and on the
use()
method, see the
documentation for the YUI Global Object.
Trigger the CSS skin
For the default "Sam" skin to apply to the Console UI, you'll
need to apply the yui3-skin-sam
class name to an element that
is a parent of the element in which the Console lives. You can usually
accomplish this simply by putting the class on the
<body>
tag:
<body class="yui3-skin-sam">
For more information on skinning YUI components and making use of default skins, see our Understanding YUI Skins tutorial.
The YUI logging subsystem
The entry point to YUI's logging subsystem is the YUI instance's log(..)
method.
// message category source Y.log("Hello world!", "info", "myapp");
If the YUI instance is configured with debug
set to
true
(the default), any calls to Y.log(..)
will
be printed to the browser's native console
if it has one, and
broadcast through the yui:log
event.
When a Console is instantiated, the native console reporting of YUI log
messages is disabled in favor of reporting in the Console UI. If you wish
to preserve native console reporting, set the
useBrowserConsole
configuration attribute to true
during Console construction.
Using the Console Widget
Instantiating and configuring a Console
Instantiation
Creating an instance of Console is very easy; there are no required configuration attributes, and it is typically rendered without reference to an existing DOM element.
YUI({..}).use('console', function (Y) { // Console has no required configuration var yconsole = new Y.Console(); yconsole.render(); // In fact, you often don't even need to store the instance new Y.Console().render(); /* YOUR CODE HERE */ });
By default, Console instances are positioned absolutely in the top right
corner of the page. As seen below and in the examples, this is configurable by
setting the style
attribute.
Common configuration attributes
Below are some common configuration attributes. Refer to the API docs for a complete list of configuration options.
Attribute | Description | Default |
---|---|---|
logLevel |
Set to "warn" or "error" to omit messages of lesser severity | "info" |
newestOnTop |
Set to false to place new messages below prior messages | true |
consoleLimit |
Limit the number of messages displayed in the UI | 300 |
height |
Specify the height of the Console. Useful for displaying more messages | "300px" |
style |
Relationship of the Console to the page content. Supported values are "inline", "block", and "separate" | "separate" (absolute positioned in the top right corner) |
The print loop
Incoming log messages are buffered and printed in a scheduled batch cycle to lessen the impact to the normal operation of the page. The print loop renders a fixed number of buffered messages at a time.
The print loop behavior can be configured with the
printTimeout
and printLimit
configuration
attributes. The former controls the millisecond timeout between iterations
of the print loop. The latter limits the number of entries to add to the
Console in each iteration of the print loop. By default their respective
values are 100 and 50.
Share a Console between YUI instances
Console behaves like any other YUI module and remains sandboxed inside the particular YUI instance that spawned it. However, it is possible to create a universal Console to report the activity in the logging subsystems of every YUI instance on the page.
To support cross YUI instance communication, a shared global EventTarget
named Y.Global
is exposed on every YUI instance, and
yui:log
events are configured to bubble to this
EventTarget.
Console has attributes logSource
and logEvent
that can be used to link the Console instance up to an entirely different
messaging subsystem. By setting the Console's logSource
to
Y.Global
and leaving the logEvent
alone, the
single Console instance will receive log messages from every YUI instance
on the page.
YUI().use('console','overlay', function (Y) { // Create a universal Console new Y.Console({ logSource: Y.Global }).render(); /* YOUR CODE HERE */ }); YUI().use('dd', function (Y) { // Y.log statements here will be reported in the Console generated in the // other YUI instance. });
Look at the Creating a universal Console example for reference.
Console display
Anatomy of the Console
The Console has a very simple display, split into a header, body, and footer.
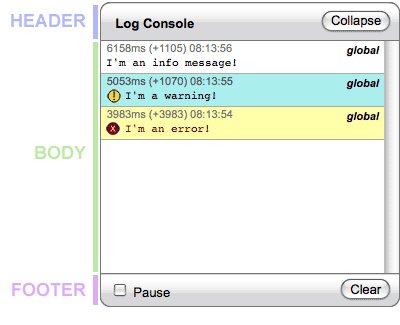
The default placement of a Console is absolutely positioned in the top
right corner of the page. This can be configured with the
style
attribute. The Creating a Console for
debugging example illustrates how to accomplish this.
Anatomy of a message
Incoming log messages are normalized to objects with the following properties:
Property | Description |
---|---|
message |
The message text |
category |
The category or log level of the message (e.g. "info", "warn", or "note") |
source |
The name of the source module |
localTime |
The time the message was received |
elapsedTime |
The time elapsed since the last message was received |
totalTime |
The time elapsed since the Console was instantiated |
These message objects are eventually rendered into the Console body like this:
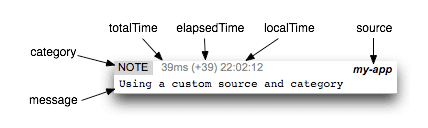
Log messages from the standard logLevel
categories
"info", "warn", and "error" get special
visual treatment. Specifically, the category is omitted from the message
meta and "warn" and "error" messages include an icon
and specific coloring.
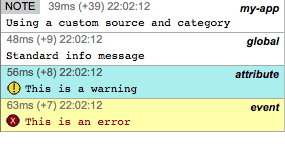
Console interaction
Collapsing, expanding, hiding and showing
Collapse and expand the Console with the Collapse/Expand button in the
header or by setting the collapsed
attribute to
true
or calling the instance's collapse()
and
expand()
methods.

Calling the instance's hide()
method will entirely remove the
UI from the page. show()
will cause it to reappear.
Pausing
Pause the Console with either the checkbox in the Console footer or by
setting the paused
attribute.
When paused, messages will accumulate in the buffer, but will not be displayed until the Console is unpaused.
Clearing and resetting
Flush the body of messages by clicking the Clear button in the Console
footer or calling the instance's clearConsole()
method.
Clearing the Console will also flush the buffered messages not yet printed.
Calling the instance's reset()
method will clear the Console,
flush the buffer, unpause, and reseed the Console's
startTime
.
Filtering Console messages
Log messages can typically be filtered in two ways: by category (or
logLevel
) and by source. Category filtering is only available
at the Console level, but source filtering can be accomplished at the YUI
config level or the Console level (via the ConsoleFilters plugin). Each approach below
has a varying degree of impact to your page's performance.
Using debug files
All YUI module files come in three flavors:
module-min.js
(min version)module.js
(raw version)module-debug.js
(debug version)
Of these, only the debug version includes Y.log(..)
statements. Explicitly including <script>
tags in your
source pointing to either the raw or min version of a module will
effectively filter out messages from that module. The combo service
supports combining any mixture of min, raw, and debug files as well.
<script src="http://yui.yahooapis.com/3.8.0/build/yui/yui-min.js"></script> <!-- Include debug messages from the node module --> <script src="http://yui.yahooapis.com/3.8.0/build/node/node-debug.js"></script> <script> YUI().use('overlay', function (Y) { // Overlay requires Node. Any other missing dependencies are automatically // loaded, excluding node-min.js since the node module is already provided // by the inline script tag. }); </script>
Th is has the least impact on page performance.
Choose which modules log
There are five YUI instance configurations that affect the logging subsystem behavior:
Property | Example | Role |
---|---|---|
debug |
Y.config.debug = false; |
If false , calls to Y.log(..) do nothing |
filter |
Y.config.filter = 'raw'; |
Set to "raw" or "debug" to specify a default version of all included modules. Default value is "min" |
filters |
|
Like filter but can be used to specify file version on a per-module basis. |
logInclude |
|
Allow only log messages assigned to the specified sources to propagate. Note a single module may use multiple sources. |
logExclude |
|
Prevent log messages from the specified sources from propagating. Typically either logInclude or logExclude is configured, not both. |
// Setting debug to true is unnecessary, but is included for illustration. // Setting filter to 'raw' facilitates stepping through module code during // debugging. Specifying 'debug' filters for slider and dd will result in all // log statements from those modules being included. YUI({ debug: true, useBrowserConsole: false, filter: 'raw', filters: { slider: 'debug', dd: 'debug' } }).use('slider', 'console', function (Y) { ... }); // Request the debug version of all loaded files, but only allow log statements // from the sources 'node' and 'dom-screen'. YUI({ useBrowserConsole: false, filter: 'debug', logInclude: { node: true, "dom-screen": true } }).use('slider', 'console', function (Y) { ... });
Specifying the filters
config to include only debug versions
of the modules you want log messages from is effectively the same as the
prior option, and has the same effect on page performance. Specifying a
"debug" filter and filtering sources via logInclude
or logExclude
has a greater impact because the calls to
Y.log(..)
are still present in all modules, even though they
will be ignored.
Look at the YUI configuration to filter log messages example for reference.
logLevel
Most log statements in YUI 3 modules are "info" messages.
Changing the logLevel
configuration for your Console instance
will limit the messages that display in the Console. Obviously, the debug
files must be used for this as well.
YUI({ useBrowserConsole: false, filter: 'debug' // use all debug files }).use('overlay', 'console', function (Y) { // Only allow 'warn' or 'error' messages to display new Y.Console({ logLevel: 'warn' }).render(); });
Console will actually default its logLevel
attribute from the
so named YUI configuration property if it is specified. Note that unlike
the attributes listed in the previous option, setting the
logLevel
in the YUI config will not alter the behavior of the
logging subsystem. The configuration is simply used as a default value for
instantiated Consoles.
// Include debug version of the dom module, but only broadcast log messages // from the 'dom-screen' source that are warnings or errors. YUI({ useBrowserConsole: false, filters: { dom: 'debug' }, logInclude: { 'dom-screen': true }, logLevel: 'warn' // this has no affect on Y.log statements }).use('dd','console', function (Y) { // With the YUI config above, these are now equivalent var consoleA = new Y.Console(); var consoleB = new Y.Console({ logLevel: 'warn' }); });
logLevel
filtering, as with any filtering occurring at the
Console, has a greater impact on page performance, since all messages,
regardless of their category, are being broadcast from the logging
subsystem to the Console before any preventative action is taken.
Preventing the Console's entry
event
Within Console, messages are transfered to the print loop buffer via an
entry
event. For fine grained control over which messages
reach the Console body, implementers can subscribe to the event and prevent
messages from being displayed by calling preventDefault()
on
the event.
var yconsole = new Y.Console(); yconsole.on('entry', function (e) { // the normalized message object is stored on the event in the 'message' // property. if (/Frank/.test(e.message.message)) { e.preventDefault(); // we don't talk about Frank here. } });
The signature of the normalized message is noted above.
This approach affords the most flexibility, as you can filter by arbitrarily complex criteria, and is the only option here not limited to comparing the category or source.
This flexibility comes at a cost, though. Because the subscriber code is necessarily executed for every log message received, there is a greater impact on page performance .
ConsoleFilters plugin
For runtime display filtering by both category and source, the ConsoleFilters plugin can be added to the
Console.
YUI({..}).use('console-filters', function (Y) { new Y.Console({ newestOnTop: false, plugins: [ Y.Plugin.ConsoleFilters ] }).render(); // OR var yconsole = new Y.Console({..}); yconsole.plug(Y.Plugin.ConsoleFilters); yconsole.render(); });
The ConsoleFilters plugin adds a set of checkboxes to the Console footer, one for each category and source currently reported. Only those messages that match one of the checked categories and sources will be displayed.
Unlike any of the prior options, messages filtered from the display by the ConsoleFilters plugin can be redisplayed by rechecking the corresponding category or source checkbox.
This approach has the greatest affect on page performance because in order to support reassembling the Console contents in real time, all Console messages are stored in memory and more conditional logic is inserted into the path from log statement to Console display.
Look at the ConsoleFilters plugin example.
Console events
In addition to the standard attributeChange
events
and others common to all Widgets, Console broadcasts the following
events:
Event | When | Payload |
---|---|---|
reset |
In response to calls to an instance's reset() method. The default function for the event performs the reset detailed above. |
none |
entry |
In response to messages being received from the yui:log . The default function for the event sends the normalized message object to the print loop buffer. |
{ message : (normalized message object) } |
This is not an exhaustive list. See the API docs for a complete listing.