Getting Started
To include the source files for ConsoleFilters Plugin and its dependencies, first load the YUI seed file if you haven't already loaded it.
<script src="http://yui.yahooapis.com/3.8.0/build/yui/yui-min.js"></script>
Next, create a new YUI instance for your application and populate it with the
modules you need by specifying them as arguments to the YUI().use()
method.
YUI will automatically load any dependencies required by the modules you
specify.
<script> // Create a new YUI instance and populate it with the required modules. YUI().use('console-filters', function (Y) { // ConsoleFilters Plugin is available and ready for use. Add implementation // code here. }); </script>
For more information on creating YUI instances and on the
use()
method, see the
documentation for the YUI Global Object.
Trigger the CSS skin
For the default "Sam" skin to apply to the Console UI, you'll
need to apply the yui-skin-sam
class name to an element that
is a parent of the element in which the Console lives. You can usually
accomplish this simply by putting the class on the
<body>
tag:
<body class="yui-skin-sam">
For more information on skinning YUI components and making use of default skins, see our Understanding YUI Skins article.
Using Plugin.ConsoleFilters
The Console Filters plugin adds a set of controls to the Console footer to allow interactive control of which log messages are displayed. It differs from other means of Console filtering by supporting display-only filtering. Messages that are filtered from view are not destroyed, and can be shown again by changing the filters.
Adding and configuring Plugin.ConsoleFilters
The Console Filters plugin has no required configuration or markup. All you need to do is plug it into your Console instance and the additional functionality will be available.
var yconsole = new Y.Console(); yconsole.plug(Y.Plugin.ConsoleFilters); // OR var yconsole = new Y.Console({ /* any other configuration */ plugins: [ Y.Plugin.ConsoleFilters ] });
Configuration
ConsoleFilters manages a category
attribute for known
categories and a source
attribute for known sources. The
value of these attributes is an object literal with an entry for each
category or source, respectively, mapping to a boolean indicating whether
or not to display associated messages.
These attributes will be updated whenever a message with an unknown
category or source is received by the Console. When a new category or
source is received, its initial visibility is set according to the value of
the defaultVisibility
attribute.
// Configure the ConsoleFilters to show attribute messages, but default all // others to hidden. var yconsole = new Y.Console({ plugins: [ { fn: Y.Plugin.ConsoleFilters, cfg: { defaultVisibility: false, source: { attribute: true } } }]}); // OR var yconsole = new Y.Console(); yconsole.plug(Y.Plugin.ConsoleFilters, { defaultVisibility: false, source: { attribute: true } });
The ConsoleFilters UI
The Console Filters plugin adds two groups of checkboxes to the Console footer. The upper set is for known categories; the lower for known sources.
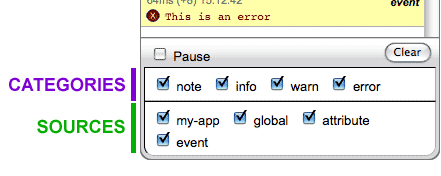
As new categories or sources are received by the Console, corresponding checkboxes are added to the respective group. You can see this in the Using the ConsoleFilters plugin example.
Interacting with the Console Filters plugin
Checking and unchecking the filter checkboxes will cause the Console body to repopulate with only those messages that match both a checked category and a checked source.
Typically, interaction with the Console Filters plugin occurs via the UI.
However, it does provide an API under the filter
namespace on
your Console instance. Through this API you can get
or
set
any of the attributes noted above or
use the following convenience methods:
hideCategory(cat1, cat2, ...catN)
showCategory(cat1, cat2, ...catN)
hideSource(src1, src2, ...srcN)
showSource(src1, src2, ...srcN)
// Setting visibility states via the attributes yconsole.filter.set('source.attribute', false); yconsole.filter.set('source.event', false); yconsole.filter.set('category.info', true); // Equivalent operations, using the convenience methods yconsole.filter.hideSource('attribute','event'); yconsole.filter.showCategory('info');
Updating the attributes via set
or any of the hide or show
methods will check or uncheck the corresponding checkbox in the UI.
Events
The Console Filters plugin does not emit any events other than the corresponding change events for its attributes.
categoryChange
sourceChange
defaultVisibilityChange