This simple tool helps you by generating code while you interactively
draw graphic paths. This was used to draw the violin example.
The Path Tool
As you drag the pencil icon, corresponding graphics code is auto-generated. This code can be copied and pasted into a graphics instance to reproduce the paths you created with the pencil.
Initial State
When the tool window is loaded, its generated code is placed in the text area control. The generated code assumes you will have a separate page to paste it into. This separate page must contain the following code: A graphics container such as this,
<div id="mygraphiccontainer"></div>
CSS such as this,
#mygraphiccontainer { position: relative; width: 700px; height:400px; }and a YUI instance containing a
Graphics
object such as this.
YUI().use('graphics', function (Y) { var mygraphic = new Y.Graphic({render:"#mygraphiccontainer"}); // generated code from the path tool goes here });
The tool generates paste-able code for an addShape
method that will
create and render a path. It generates some fill and stroke placeholder code.
You can change this to change the attributes of the fill and stroke
after it's pasted into your code.
Note: As you draw paths with the pencil, they won't have these attributes; you'll only see thin, red lines for precision. The placeholder attributes below will only show after you paste the code in your page.
{ type: "path", stroke: { weight: 2, color: "#00dd00" }, fill: { type: "linear", stops: [ {color: "#cc0000", opacity: 1, offset: 0}, {color: "#00cc00", opacity: 0.3, offset: 0.8} // Opacity of stops not available in IE ] } }
LineTo (Drag Pencil)
Drag the pencil icon. The drag:end
event sets the lineTo
XY value.
MoveTo (Shift + Drag)
Drag the pencil icon with the shift key down to move without drawing a line.
CurveTo (Alt + Drag)
Drag the pencil icon with the alt key down. This draws a curve and displays
two draggable control points. The curve adjusts while you drag the control points.
Clicking the pencil icon, or starting another line, finalizes the curveTo
.
New Path Object
Crate a new path object with a new name by changing the name in the 'Graphic Object Name' text input, then clicking the 'New' button.
Trace an Image
You can modify the CSS of #mygraphiccontainer to include an image background. This will allow you to trace the contours of an image.
#mygraphiccontainer { background: url(assets/images/my-dog.jpg); }
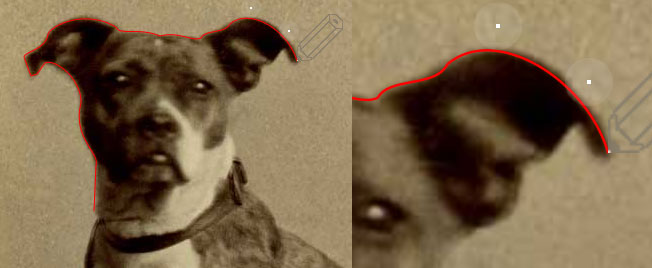