The Color Utility will give you the ability to convert colors to and from RGB and Hex. Color also provides submodules to convert to and from HSL with color-hsl
and HSV with color-hsv
.
Y.Color
also provides a very useful way to find colors that work together. color-harmony
provides the ability to get harmony colors such as complementary, triads, analogous, and more! You can even match a color to the perceived brightness of another color.
Getting Started
To include the source files for Color and its dependencies, first load the YUI seed file if you haven't already loaded it.
<script src="http://yui.yahooapis.com/3.8.0/build/yui/yui-min.js"></script>
Next, create a new YUI instance for your application and populate it with the
modules you need by specifying them as arguments to the YUI().use()
method.
YUI will automatically load any dependencies required by the modules you
specify.
<script> // Create a new YUI instance and populate it with the required modules. YUI().use('color-base', function (Y) { // Color is available and ready for use. Add implementation // code here. }); </script>
For more information on creating YUI instances and on the
use()
method, see the
documentation for the YUI Global Object.
Using Color
Color allows you to convert a color from and to hexadecimal and RGB. Color conversion methods take a string value and return a string value.
Basic Conversion
Color takes color values as strings. Color values may consist of hexadecimal "#ff00ff"
, RGB "rgb(255, 0, 255)"
, or RGBA "rgba(255, 0, 255, 1)"
.
As with taking in these values, you can also convert to any of these values.
var color = 'f00'; Y.Color.toHex(color); // #ff0000 Y.Color.toRGB(color); // rgb(255, 0, 0) Y.Color.toRGBA(color); // rgba(255, 0, 0, 1)
Color also provides a sugar method, convert()
. It takes color value as the first param and the to
type as the second value.
var color = 'f00'; Y.Color.convert(color, Y.Color.TYPES.HEX); // #ff0000 Y.Color.convert(color, Y.Color.TYPES.RGB); // rgb(255, 0, 0) Y.Color.convert(color, Y.Color.TYPES.RGBA); // rgba(255, 0, 0, 1)
Working with Arrays
If you wish to get the values back in an array, you can use the toArray
method.
Y.Color.toArray('f00'); // ['ff', '00', '00'] Y.Color.toArray('rgb(255, 0, 0)'); // [255, 0, 0] Y.Color.toArray('rgba(255, 0, 0, 1)'); // [255, 0, 0, 1]
Color also provides an easy way to convert from an array to a color string value with fromArray
. fromArray
takes an Array as the first param and template string as the second param.
There are default templates defined in Color for you to use.
Y.Color.fromArray(['ff', '00', '00'], Y.Color.TYPES.HEX); // #ff0000 Y.Color.fromArray([255, 0, 0], Y.Color.TYPES.RGB); // rgb(255, 0, 0) Y.Color.fromArray([255, 0, 0, 1], Y.Color.TYPES.RGBA); // rgb(255, 0, 0, 1)
HSL Color
You can get access to HSL color values and conversion methods by using the color-hsl
submodule.
var color = 'f00'; // direct conversion methods Y.Color.toHSL(color); // hsl(0, 100%, 50%) Y.Color.toHSLA(color); // hsla(0, 100%, 50%, 1) // convertion sugar withconvert()
andto
specification Y.Color.convert(color, Y.Color.TYPES.HSL); // hsl(0, 100%, 50%) Y.Color.convert(color, Y.Color.TYPES.HSLA); // hsla(0, 100%, 50%, 1) // convert string to an array Y.Color.toArray('hsl(0, 100%, 50%)'); // [0, 100, 50] Y.Color.toArray('hsla(0, 100%, 50%, 1)'); // [0, 100, 50, 1] // convert to string from an array Y.Color.fromArray([0, 100, 50], Y.Color.TYPES.HSL); // hsl(0, 100%, 50%) Y.Color.fromArray([0, 100, 50, 1], Y.Color.TYPES.HSLA); // hsl(0, 100%, 50%, 1)
HSV Color
You can get access to HSV color values and conversion methods by using the color-hsv
submodule.
By default, hsv strings are represented as hsv( hue, saturation%, value%)
even though these are not directly supported with CSS as hsl strings are.
var color = 'f00'; // direct conversion methods Y.Color.toHSV(color); // hsv(0, 100%, 100%) Y.Color.toHSVA(color); // hsva(0, 100%, 100%, 1) // convertion sugar withconvert()
andto
specification Y.Color.convert(color, Y.Color.TYPES.HSV); // hsv(0, 100%, 100%) Y.Color.convert(color, Y.Color.TYPES.HSVA); // hsva(0, 100%, 100%, 1) // convert string to an array Y.Color.toArray('hsv(0, 100%, 100%)'); // [0, 100, 100] Y.Color.toArray('hsva(0, 100%, 100%, 1)'); // [0, 100, 100, 1] // convert to string from an array Y.Color.fromArray([0, 100, 100], Y.Color.TYPES.HSV); // hsv(0, 100%, 100%) Y.Color.fromArray([0, 100, 100, 1], Y.Color.TYPES.HSVA); // hsv(0, 100%, 100%, 1)
Harmony Colors
Color also makes it easy to get complementary, triads, tetrads, or offsets of a color through color-harmony
. Harmony methods that return colors, will return an Array with the color provided (converted, if requested) as the first index.
<script> // Create a new YUI instance and populate it with the required modules. YUI().use('color-harmony', function (Y) { // Color is available and ready for use with harmony methods added on. // Add implementation code here. }); </script>
Color harmony methods can also do a conversion for you on the colors that are returned with the optional to
param. When no to
value is provided, the return values will match the string type initially provided.
For the following examples, we will use these color options to keep the examples easy to read.
var white = "#ffffff", black = "#000000", red = "#ff0000", orange = "#ff7700", yellow = "#ffff00", green = "#00ff00", blue = "#0000ff", purple = "#ff00ff";
Complementary
A complementary color is the opposite color on the subtractive color wheel.
getComplementary
will return an Array of two color values. The first being the color provided. The second being the complementary color.
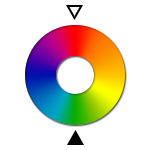
// getComplementary(color, to) Y.Color.getComplementary(red); // [ "#ff0000", "#00ff00"]
Split Complementary
Split complementary is similar to complementary, with the exception of getting one color opposite the starting color, you get two colors adjacent to the complementary color.
getSplit
will return an Array of three color values. The first being the color provided. The second being a 30 degree offset from the complementary color. The third being a -30 degree offset from the complementary color.
If you wish, you can provide a custom offset distance as the second parameter.
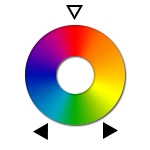
// getSplit(color, offset, to) Y.Color.getSplit(red); //["#ff0000", "#00ffff", "#55ff00"]
Analogous
Analogous colors are colors adjacent to each other. Color will return an Array of colors.
The first color is the color you provided. The next to colors are clockwise from the start color. The last two are counter clockwise from the start color.
The offset distance can be set with a Number
representing the degree between each color as the second parameter. The offset by default is 10
.
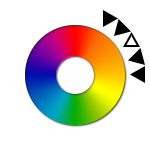
// getAnalogous(color, offset, to) Y.Color.getAnalogous(orange); // ["#ff7700", "#ff9100", "#ffae00", "#ff5900", "#ff3c00"]
Triad
A triad will return three evenly spaced colors. The first being the color provided, the next two will be 120 degree offsets clockwise.
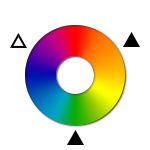
// getTriad(color, to) Y.Color.getTriad(purple); // ["#ff00ff", "#ffaa00", "#00ff00"]
Square
A square will return four evenly spaced colors. The first being the color provided, the remaining three will be 90 degree offsets clockwise.
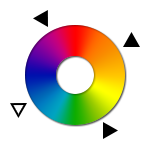
// getSquare(color, to) Y.Color.getSquare(blue); // ["#0000ff", "#ff0080", "#ffaa00", "#55ff00"]
Tetrad
Tetrad is similar to square although the offsets are not equal. Tetrad is comprised of the complementary color and an offset from the original and the complementary color.
The offset distance can be set with a Number
representing the degree between each color as the second parameter. The offset by default is 60
.
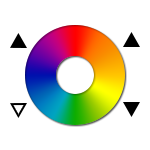
// getTetrad(color, offset, to) Y.Color.getTetrad(blue); // ["#0000ff", "#ff00ff", "#ffaa00", "#aaff00"]
Monochromatic
A monochromatic harmony set takes the hue and saturation of the provided color and returns an Array of colors with adjusted luminance from zero to one hundred percent.
The number of colors returned can be set with a Number
specifying the total number of items to be returned as the second parameter. The default is 5
resulting in five items returned.

// getMonochrome(color, count, to) Y.Color.getMonochrome(green); // ["#000000", "#008000", "#00ff00", "#80ff80", "#ffffff"]
Similar Colors
You can also get a set of colors similar to provided color. A similar color is a color with a similar hue, saturation, and/or luminance.
To change the maximum offset, set the second param with a Number. The offset by default is 10
.
You can specify the number of similar colors returned with the third param as a Number. By default 5
similar colors are returned in addition to the provided color.
// getSimilar(color, offset, count, to) Y.Color.getSimilar(purple); // ["#ff00ff", "#f429ff", "#bb03d3", "#c405d6", "#e01ff9", "#e402e8"]
Hue, Saturation, Luminance Offsets
You adjust hue, saturation, or luminance individually using getOffset
. The offset is plus or minus the current value. Saturation and luminance can only go as high as 100
and as low as 0
.
To set the amount to adjust, supply an Object as the second parameter. This object may contain any combination of h
, s
, and l
representing hue, saturation, and luminance respectively.
// getOffset(color, adjust, to) Y.Color.getOffset(red, {h: 40}); // #ff6e00 Y.Color.getOffset(red, {l: 20}); // #ff6666 Y.Color.getOffset(red, {s: -50}); // #bf4040
You can also offset multiple factors at the same time.
Y.Color.getOffset(red, {h: 40, l: 20, s: -50}); // #d9ad8c
Perceived Brightness
Different hues at the same saturation and luminance can be perceived brighter or darker than each other (such as blue and yellow). Brightness is represented as a value from 0
to 100
— where 0
is darkest (black) and 100
is brightest (white).
Note: getBrightness
uses a weighted distance in 3D space algorithm. Traditionally these values are {r: 0.241, g: 0.691, b: 0.068 }
. These values were adjusted during tests to { r: 0.221, g: 0.711, b: 0.068 }
. You can override these values with Y.Color._brightnessWeights
.
// getBrightness(color) Y.Color.getBrightness(white); // 100 Y.Color.getBrightness(black); // 0 Y.Color.getBrightness(yellow); // 97
Match Brightness
You may wish to match one color to the preceived brightness of another color. This process will keep the hue and saturation the same, and only adjust the luminance to a color that is the same, or nearly the same, perceived brightness.
getSimiliarBrightness
takes two parameters. The first is the color you wish to adjust. The second is the color to which you wish to match the perceived brightness.
It will return a single color value string.
//getSimilarBrightness(color, match, to) Y.Color.getSimilarBrightness(orange, blue); // adjust orange to match the brightness of blue, returns #703800 Y.Color.getSimilarBrightness(white, green); // adjust white to match the brightness of green, returns #d9d9d9